Assume that a bank maintains two kinds of accounts for customers, one called as savings and the other as current account. The savings account provides compound interest and withdrawal facilities but no cheque book facility. The current account provides cheque book facility but no interest. Current account holders should also maintain a minimum balance and if the balance falls below this level a service charge is imposed.
Create a class account that stores customer name, account number and type of account. From this derive the classes cur_acct and sav_acct to make them more specific to their requirements. Include necessary member functions in order to achieve the following tasks:
- (a) Accept the deposit from a customer and update the balance.
- (b) Display the balance.
- (c) Compute and deposit interest.
- (d) Permit withdrawal and update the balance.
- (e) Check for the minimum balance, impose penalty, necessary and update the balance.
- Do not use any constructors. Use member functions to initialize class members.
#include<iostream> #include<stdio> #include<string> #include<math> #define minimum 500 #define service_charge 100 #define r 0.15 using namespace std; class account { protected: char name[100]; int ac_number; char ac_type[100]; public: void creat( char *t); }; void account::creat(char *t) { cout<<" Enter customer name :"; gets(name); strcpy(ac_type,t); cout<<" Enter account number :"; cin>>ac_number; } class cur_acct: public account { private: float balance; public: void deposite(float d); void withdraw(float w); void display(); }; void cur_acct::deposite(float d) { balance=d; } void cur_acct::withdraw(float w) { if(balance<w) cout<<" sorry your balance is less than your withdrawal amount \n"; else { balance-=w; if(balance<minimum) cout<<"\n your current balance is :"<<balance<<" which is less than"<<minimum<<"\n your account is discharged by "<<service_charge<<"TK \n"<<" You must store "<<minimum<<"TK to avoid discharge \n "<<" Do you want to withdraw ? press 1 for yes press 0 for no \n"<<" what is your option ?"; int test; cin>>test; if(test==0) balance+=w; } } void cur_acct::display() { cout<<"\n Now balance = "<<balance<<"\n"; } class sav_acct:public account { float balance; int d,m,y; public: void deposite(float d); void withdraw(float w); void display(); void set_date(int a,int b,int c){d=a;m=b;y=c;} void interest(); }; void sav_acct::deposite(float d) { int x,y,z; cout<<" Enter today's date(i,e day,month,year) : "; cin>>x>>y>>z; set_date(x,y,z); balance=d; } void sav_acct::withdraw(float w) { if(balance<w) cout<<" sorry your balance is less than your withdrawal amount \n"; else { balance-=w; if(balance<minimum) { cout<<"\n your current balance is :"<<balance<<" which is less than"<<minimum<<"\n your account is discharged by "<<service_charge<<"TK \n"<<" You must store "<<minimum<<"TK to avoid discharge \n "<<" Do you want to withdraw ? press 1 for yes press 0 for no \n"<<" what is your option ?"; int test; cin>>test; if(test==0) balance+=w; } } } void sav_acct::display() { cout<<"\n Now balance = "<<balance; } void sav_acct::interest() { int D[12]={31,28,31,30,31,30,31,31,30,31,30,31}; int d1,y1,m1; cout<<" Enter today's date :(i,e day,month,year) "; cin>>d1>>m1>>y1; int iday,fday; iday=d; fday=d1; for(int i=0;i<m1;i++) { fday+=D[i]; } for(i=0;i<m;i++) { iday+=D[i]; } int tday; tday=fday-iday; float ty; ty=float(tday)/365+y1-y; float intrst; intrst=ty*r*balance; cout<<" Interest is : "<<intrst<<"\n"; balance+=intrst; } int main() { sav_acct santo; santo.creat("savings"); float d; cout<<" Enter your deposit amount : "; cin>>d; santo.deposite(d); santo.display(); int t; cout<<"\n press 1 to see your interest : \n" <<" press 0 to skip : "; cin>>t; if(t==1) santo.interest(); cout<<"\n Enter your withdrawal amount :"; float w; cin>>w; santo.withdraw(w); santo.display(); return 0; }
OUTPUT
Enter customer name :Rimo
Enter account number :10617
Enter your deposit amount : 10000
Enter today’s date(i,e day,month,year) : 13 7 2010
Now balance = 10000
press 1 to see your interest :
press 0 to skip : 1
Enter today’s date :(i,e day,month,year) 15 8 2010
Interest is : 135.61644
Enter your withdrawal amount :500
Now balance = 9635.616211
Modify the program of exercise 8.1 to include constructors for all three classes.
#include<iostream> #include<stdio> #include<string> #include<math> #define minimum 500 #define service_charge 100 #define r 0.15 using namespace std; class account { protected: char name[100]; int ac_number; char ac_type[100]; public: account( char *n,char *t,int no); }; account::account(char *n,char *t,int no) { strcpy(name,n); strcpy(ac_type,t); ac_number=no; } class cur_acct: public account { private: float balance,d,w; public: void withdraw(float ww); void deposit(float d){balance=d;} cur_acct(char *n,char *t,int number,float dp,float wd): account(n,t,number) { d=dp; w=wd; deposit(d); withdraw(w); } void display(); }; void cur_acct::withdraw(float ww) { if(balance<ww) cout<<" sorry your balance is less than your withdrawal amount \n"; else { balance-=ww; if(balance<minimum) { cout<<"\n your current balance is :"<<balance<<" which is less than"<<minimum<<"\n your account is discharged by "<<service_charge<<"TK \n"<<" You must store "<<minimum<<"TK to avoid discharge \n "<<" Do you want to withdraw ? press 1 for yes press 0 for no \n"<<" what is your option ?"; int test; cin>>test; if(test==0) balance+=w; } else ; } } void cur_acct::display() { cout<<"\n Now balance = "<<balance<<"\n"; } class sav_acct:public account { float balance; int d,m,y; public: void deposite(float d){balance=d;set_date();} void withdraw(float w); void display(); void set_date(){d=12;m=1;y=2010;} void interest(); sav_acct(char *n,char *t,int number,float dp,float wd): account(n,t,number) { float d,w; d=dp; w=wd; deposite(d); interest(); withdraw(w); } }; void sav_acct::withdraw(float w) { if(balance<w) cout<<" sorry your balance is less than your withdrawal amount \n"; else { balance-=w; if(balance<minimum) { cout<<"\n your current balance is :"<<balance<<" which is less than"<<minimum<<"\n your account is discharged by "<<service_charge<<"TK \n"<<" You must store "<<minimum<<"TK to avoid discharge \n "<<" Do you want to withdraw ? press 1 for yes press 0 for no \n"<<" what is your option ?"; int test; cin>>test; if(test==0) balance+=w; } else ; } } void sav_acct::display() { cout<<"\n Now balance = "<<balance; } void sav_acct::interest() { int D[12]={31,28,31,30,31,30,31,31,30,31,30,31}; int d1,y1,m1; cout<<" Enter today's date :(i,e day,month,year) "; cin>>d1>>m1>>y1; int iday,fday; iday=d; fday=d1; for(int i=0;i<m1;i++) { fday+=D[i]; } for(i=0;i<m;i++) { iday+=D[i]; } int tday; tday=fday-iday; float ty; ty=float(tday)/365+y1-y; balance=balance*pow((1+r),ty); } int main() { float d; cout<<" Enter customer name :"; char name[100]; gets(name); cout<<" Enter account number :"; int number; cin>>number; cout<<" Enter your deposit amount : "; cin>>d; cout<<" Enter your withdrawal amount :"; float w; cin>>w; //cur_acct s("current",name,number,d,w); //s.display(); sav_acct c("savings",name,number,d,w); c.display(); return 0; }
OUTPUT
Enter customer name :mehedi
Enter account number :1457
Enter your deposit amount : 5000
Enter your withdrawal amount :1200
Enter today’s date :(i,e day,month,year) 13 7 2010
Now balance = 4160.875977
An educational institution wishes to maintain a database of its employees. The database is divided into a number of classes whose hierarchical relationships are shown in following figure. The figure also shows the minimum information required for each class. Specify all classes and define functions to create the database and retrieve individual information as and when required.
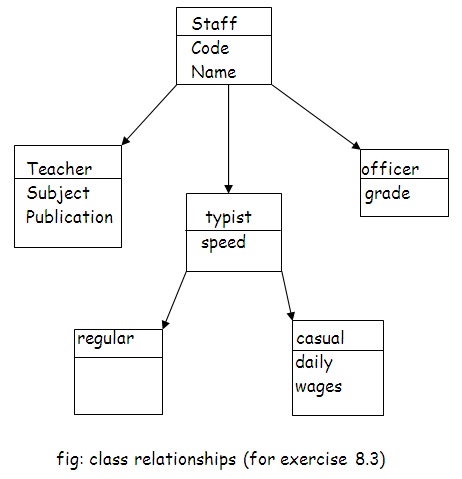
#include<iostream> #include<iomanip> #include<string> using namespace std; class staff { public: int code; char name[100]; public: void set_info(char *n,int c) { strcpy(name,n); code=c; } }; class teacher : public staff { protected: char sub[100],publication[100]; public: void set_details(char *s,char *p) { strcpy(sub,s);strcpy(publication,p); } void show() { cout<<"name"<<setw(8)<<"code"<<setw(15)<<"subject"<<setw(25) <<"publication"<<endl<<name<<setw(8)<<code<<setw(25)<<sub<<setw(22)<<publication<<endl; } }; class officer:public staff { char grade[100]; public: void set_details(char *g) { strcpy(grade,g); } void show() { cout<<" name "<<setw(15)<<"code"<<setw(15)<<"Category "<<endl <<name<<setw(10)<<code<<setw(15)<<grade<<endl; } }; class typist: public staff { protected: float speed; public: void set_speed(float s) { speed=s; } }; class regular:public typist { protected: float wage; public: void set_wage(float w){wage=w;} void show() { cout<<"name"<<setw(16)<<"code"<<setw(15)<<"speed"<<setw(15) <<"wage"<<endl<<name<<setw(10)<<code<<setw(15)<<speed <<setw(15)<<wage<<endl; } }; class causal:public typist { float wage; public: void set_wage(float w){wage=w;} void show() { cout<<"name"<<setw(16)<<"code"<<setw(15)<<"speed"<<setw(15) <<"wage"<<endl<<name<<setw(10)<<code<<setw(15)<<speed <<setw(15)<<wage<<endl; } }; int main() { teacher t; t.set_info("Ataur",420); t.set_details("programming with c++"," Tata McGraw Hill"); officer o; o.set_info("Md. Rashed",222); o.set_details("First class"); regular rt; rt.set_info("Robiul Awal",333); rt.set_speed(85.5); rt.set_wage(15000); causal ct; ct.set_info("Kawser Ahmed",333); ct.set_speed(78.9); ct.set_wage(10000); cout<<" About teacher: "<<endl; t.show(); cout<<" About officer:"<<endl; o.show(); cout<<" About regular typist :"<<endl; rt.show(); cout<<" About causal typist :"<<endl; ct.show(); return 0; }
OUTPUT
About teacher:
name code subject publication
Ataur 420 programming with c++ Tata McGraw Hill
About officer:
name code Category
Md. Rashed 222 First class
About regular typist :
name code speed wage
Robiul Awal 333 85.5 15000
About causal typist :
name code speed wage
Kawser Ahmed 333 78.900002 10000
The database created in exercise 8.3 does not include educational information of the staff. It has been decided to add this information to teachers and officers (and not for typists) which will help management in decision making with regard to training, promotions etc. Add another data class called education that holds two pieces of educational information namely highest qualification in general education and highest professional qualification. This class should be inherited by the classes teacher and officer. Modify the program of exercise 8.19 to incorporate these additions.
#include<iostream> #include<iomanip> #include<string> using namespace std; class staff { protected: int code; char name[100]; public: void set_info(char *n,int c) { strcpy(name,n); code=c; } }; class education:public staff { protected: char quali[100]; public: void set_qualification(char *q){strcpy(quali,q);} }; class teacher : public education { protected: char sub[100],publication[100]; public: void set_details(char *s,char *p) { strcpy(sub,s);strcpy(publication,p); } void show() { cout<<" name "<<setw(8)<<"code"<<setw(15) <<"subject"<<setw(22)<<"publication" <<setw(25)<<"qualification"<<endl <<name<<setw(8)<<code<<setw(25) <<sub<<setw(18)<<publication<<setw(25)<<quali<<endl; } }; class officer:public education { char grade[100]; public: void set_details(char *g) { strcpy(grade,g); } void show() { cout<<" name "<<setw(15)<<"code"<<setw(15)<<"Catagory " <<setw(22)<<"Qualification"<<endl<<name<<setw(10) <<code<<setw(15)<<grade<<setw(25)<<quali<<endl<<endl; } }; class typist: public staff { protected: float speed; public: void set_speed(float s) { speed=s; } }; class regular:public typist { protected: float wage; public: void set_wage(float w){wage=w;} void show() { cout<<" name "<<setw(16)<<"code"<<setw(15)<<"speed" <<setw(15)<<"wage"<<endl<<name<<setw(10)<<code <<setw(15)<<speed<<setw(15)<<wage<<endl<<endl; } }; class causal:public typist { float wage; public: void set_wage(float w){wage=w;} void show() { cout<<" name "<<setw(16)<<"code"<<setw(15)<<"speed" <<setw(15)<<"wage"<<endl<<name<<setw(10)<<code <<setw(15)<<speed<<setw(15)<<wage<<endl<<endl; } }; int main() { teacher t; t.set_info("Ataur",420); t.set_details("programming with c++"," Tata McGraw Hill"); t.set_qualification("PHD from programming "); officer o; o.set_info("Md. Rashed",222); o.set_details("First class"); o.set_qualification("2 years experienced"); regular rt; rt.set_info("Robiul Awal",333); rt.set_speed(85.5); rt.set_wage(15000); causal ct; ct.set_info("Kawser Ahmed",333); ct.set_speed(78.9); ct.set_wage(10000); cout<<" About teacher: "<<endl; t.show(); cout<<" About officer:"<<endl; o.show(); cout<<" About regular typist :"<<endl; rt.show(); cout<<" About causal typist :"<<endl; ct.show(); return 0; }
OUTPUT
About teacher:
name code subject publication qualification
Ataur 420 programming with c++ Tata McGraw Hill PHD from programming-
About officer:
name code Catagory Qualification
Md. Rashed 222 First class 2 years experienced
About regular typist :
name code speed wage
Robiul Awal 333 85.5 15000
About causal typist :
name code speed wage
Kawser 333 78.900002 10000
Consider a class network of the following figure. The class master derives information from both account and admin classes which in turn derives information from the class person. Define all the four classes and write a program to create, update and display the information contained in master objects.
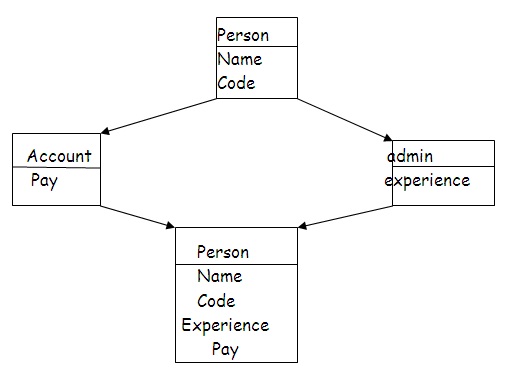
#include<iostream> #include<iomanip> #include<string> using namespace std; class staff { protected: int code; char name[100]; public: void set_info(char *n,int c) { strcpy(name,n); code=c; } }; class education:public staff { protected: char quali[100]; public: void set_qualification(char *q){strcpy(quali,q);} }; class teacher : public education { protected: char sub[100],publication[100]; public: void set_details(char *s,char *p) { strcpy(sub,s);strcpy(publication,p); } void show() { cout<<"name"<<setw(8)<<"code"<<setw(15)<<"subject"<<setw(22) <<"publication"<<setw(25)<<"qualification"<<endl<<name<<setw(8) <<code<<setw(25)<<sub<<setw(18)<<publication<<setw(25)<<quail <<endl; } }; class officer:public education { char grade[100]; public: void set_details(char *g) { strcpy(grade,g); } void show() { cout<<"name"<<setw(15)<<"code"<<setw(15)<<"Catagory" <<setw(22)<<"Qualification"<<endl<<name<<setw(10) <<code<<setw(15)<<grade<<setw(25)<<quali<<endl<<endl; } }; class typist: public staff { protected: float speed; public: void set_speed(float s) { speed=s; } }; class regular:public typist { protected: float wage; public: void set_wage(float w){wage=w;} void show() { cout<<"name"<<setw(16)<<"code"<<setw(15)<<"speed"<<setw(15) <<"wage"<<endl<<name<<setw(10)<<code<<setw(15)<<speed <<setw(15)<<wage<<endl<<endl; } }; class causal:public typist { float wage; public: void set_wage(float w){wage=w;} void show() { cout<<"name"<<setw(16)<<"code"<<setw(15)<<"speed"<<setw(15) <<"wage"<<endl<<name<<setw(10)<<code<<setw(15)<<speed <<setw(15)<<wage<<endl<<endl; } }; int main() { teacher t; t.set_info("Ataur",420); t.set_details("programming with c++"," Tata McGraw Hill"); t.set_qualification("PHD from programming "); officer o; o.set_info("Md. Rashed",222); o.set_details("First class"); o.set_qualification("2 years experienced"); regular rt; rt.set_info("Robiul Awal",333); rt.set_speed(85.5); rt.set_wage(15000); causal ct; ct.set_info("Kawser Ahmed",333); ct.set_speed(78.9); ct.set_wage(10000); cout<<" About teacher: "<<endl; t.show(); cout<<" About officer:"<<endl; o.show(); cout<<" About regular typist :"<<endl; rt.show(); cout<<" About causal typist :"<<endl; ct.show(); return 0; }
OUTPUT
name code Experience payment
Hasibul 111 3 years 1500tk
In exercise 8.3 the classes teacher, officer, and typist are derived from the class staff. As we know we can use container classes in place of inheritance in some situations. Redesign the program of exercise 8.3 such that the classes teacher, officer and typist contain the objects of staff.
#include<iostream> #include<iomanip> #include<string> using namespace std; class staff { public: int code; char name[100]; public: void set_info(char *n,int c) { strcpy(name,n); code=c; } }; class teacher : public staff { protected: char sub[100],publication[100]; public: void set_details(char *s,char *p) { strcpy(sub,s);strcpy(publication,p); } void show() { cout<<"name"<<setw(8)<<"code"<<setw(15)<<"subject"<<setw(25) <<"publication"<<endl<<name<<setw(8)<<code<<setw(25)<<sub <<setw(22)<<publication<<endl; } }; class officer:public staff { char grade[100]; public: void set_details(char *g) { strcpy(grade,g); } void show() { cout<<" name "<<setw(15)<<"code"<<setw(15)<<"Catagory "<<endl <<name<<setw(10)<<code<<setw(15)<<grade<<endl; } }; class typist: public staff { protected: float speed; public: void set_speed(float s) { speed=s; } void show() { cout<<" name "<<setw(15)<<"code"<<setw(15)<<"speed"<<endl <<name<<setw(10)<<code<<setw(15)<<speed<<endl<<endl; } }; int main() { teacher t; t.set_info("Ataur",420); t.set_details("programming with c++"," Tata McGraw Hill"); officer o; o.set_info("Md. Rashed",222); o.set_details("First class"); typist tp; tp.set_info("Robiul Awal",333); tp.set_speed(85.5); cout<<" About teacher: "<<endl; t.show(); cout<<" About officer:"<<endl; o.show(); cout<<" About typist :"<<endl; tp.show(); return 0; }
OUTPUT
About teacher:
name code subject publication
Ataur 420 programming with c++ Tata McGraw Hill
About officer:
name code Catagory
Md. Rashed 222 First class
About typist :
name code speed
Robiul Awal 333 85.5
We have learned that OOP is well suited for designing simulation programs. Using the techniques and tricks learned so far, design a program that would simulate a simple real-world system familiar to you
#include<iostream> #include<stdio> #include<string> #include<iomanip> #include<conio> using namespace std; char *sub[10]={"Bangla 1st paper","Bangla 2nd paper","English 1st paper", "English 2nd paper","Mathematics","Religion", "Physics","Chemistry","Sociology","Higher Mathematics"}; class student_info { public: char name[40]; char roll[20]; public: void set_info(); }; void student_info::set_info() { cout<<"Enter student name : "; gets(name); cout<<"Enter roll: "; gets(roll); } class subject :public student_info { public: float mark[10]; public: void set_mark(); }; void subject::set_mark() { cout<<" marks of : \n"; for(int i=0;i<10;i++) { cout<<sub[i]<<" = ? "; cin>>mark[i]; } } class conversion :public subject { float gpa[10]; char grade[20][20]; public: void convert_to_gpa(); void show(); }; void conversion::convert_to_gpa() { for(int i=0;i<10;i++) { if(mark[i]>=80) { gpa[i]=5.00; strcpy(grade[i],"A+"); } else if(mark[i]>=70 && mark[i]<80) { gpa[i]=4.00; strcpy(grade[i],"A"); } else if(mark[i]>=60 && mark[i]<70) { gpa[i]=3.50; strcpy(grade[i],"A-"); } else if(mark[i]>=50 && mark[i]<60) { gpa[i]=3.00; strcpy(grade[i],"B"); } else if(mark[i]>=40 && mark[i]<50) { gpa[i]=2.00; strcpy(grade[i],"C"); } else if(mark[i]>=33 && mark[i]<40) { gpa[i]=1.00; strcpy(grade[i],"D"); } else { gpa[i]=0.00; strcpy(grade[i],"Fail"); } } } void conversion::show() { cout<<" result of \n"; cout<<"name :"<<name<<"\n"; cout<<"Roll : "<<roll<<"\n"; cout<<setw(25)<<"Subject"<<setw(17)<<"Marks" <<setw(14)<<"GPA"<<setw(12)<<"Grade \n"; for(int i=0;i<10;i++) { cout<<setw(25)<<sub[i]<<setw(15)<<mark[i] <<setw(15)<<gpa[i]<<setw(10)<<grade[i]<<"\n"; } } int main() { clrscr(); conversion A; A.set_info(); A.set_mark(); A.convert_to_gpa(); A.show(); getch(); return 0; }
OUTPUT
Enter student name : santo
Enter roll: 156271
marks of :
Bangla 1st paper = ? 74
Bangla 2nd paper = ? 87
English 1st paper = ? 45
English 2nd paper = ? 56
Mathematics = ? 87
Religion = ? 59
Physics = ? 75
Chemistry = ? 65
Sociology = ? 39
Higher Mathematics = ? 86
result of
name :santo
Roll : 156271
Subject Marks GPA Grade
Bangla 1st paper 74 4 A
Bangla 2nd paper 87 5 A+
English 1st paper 45 2 C
English 2nd paper 56 3 B
Mathematics 87 5 A+
Religion 59 3 B
Physics 75 4 A
Chemistry 65 3.5 A-
Sociology 39 1 D
Higher Mathematics 86 5 A+
Next Previous