What does inheritance mean in C++?
The mechanism of deriving a new class from an old one is called inheritance. The old class is referred to as the base class and the new one is called derived class.
What are the different forms of inheritance? Give an example for each.
Different forms of inheritence:
1. Single inheritence : Only one derived class inherited from only one base class is called single inheritence.
Example: Let A is a base class
and B is a new class derived from A

This is written in program as following:
class A {……….};
class B : Public A {……..};
2.Multiple inheritence : A class can inherit the attributes of two or more classes. This is known as multiple inheritence.
Example :
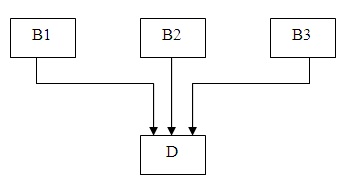
Class D : visibilityB1, visibility.B2, visibility B3
{ (Body of D) }
* visibility may be public or private.
3.Multilevel inheritance : It a class is derived from a base class, and another class is derived from this derived class and so on, then it is called multilevel inheritance.
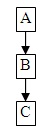
Example:
Class A { ….. };
Class B : Public A { ….. };
Class C : Private B{ ….. };
4.Hierarchical inheritance: It the inheritance follows the hierarchical design of a program, then it is called hierarchical inheritance.
Example :
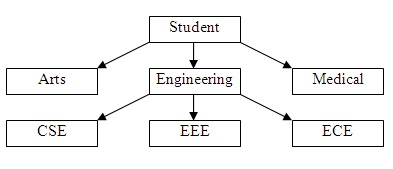
This design is implemented in program as follows:
Class student { …… }; // base class,
Class Arts : Public student { ……};
Class Medical : Public student {…….};
Class Engineering : Public student { ……};
Class CSE : Public Engineering { ……. };
Class EEE : Public Engineering { …… };
Class ECE : Public Engineering { …… };
* here all inheritance are considered as public you can private inheritance also. as you wish.
5.Hybrid inheritance: When multi level and multiple inheritances are applied to an inheritance, then it is called Hybrid inheritance.
Example :
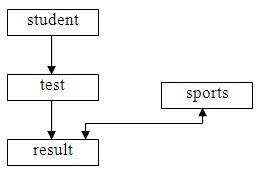
In program :
Class student {……};
Class test : public student {……};
Class result : public test {…….};
Class result : public sports {…….};In program :
Class student {……};
Class test : public student {……};
Class result : public test {…….};
Class result : public sports {…….};
Describe the syntax of the single inheritance in C++.
Syntax of single inheritance:
Class Derived name : visibility mode Base_class name
{ Body of derived class };
* visibility mode may be public or private.
or protected
We know that a private member of a base class is not inheritable. Is it anyway possible for the objects of a derived class to access the private members of the base class? If yes, how? Remember, the base class cannot be modified.
Yes. It is possible for the objects of derived class to access the private member of the base class by a member function of base class which is public. The following example explains this :
#include<iostream> using namespace std; class B { int a; // a is private that can not be inherited. public: int get_a(); void set_a(); }; class D:public B { int b; public: void display_a(); }; void D :: display_a() { cout<<" a = "<<get_a()<<"\n"; // a is accessed by member function get_a(). } void B :: set_a() { a=156271; } int B :: get_a() { return a; } void main() { D d; d.set_a(); d.display_a(); }
How do the properties of the following two derived classes differ?
- (a) class D1: private B(// ….);
- (b) class D2: public B(//….);
- (a) Private member of B can not be inherited in D1 Protected member of B become private in D1 public member of B become private in D1.
- (b) Private member of B can not be inherited in D2 Protected member of B remains protected in D2 Public member of B remains public in D2
When do we use the protected visibility specifier to a class member?
When we want access a data member by the member function within its class and by the member functions immediately derived from it, then we use visibility modifier protected.
Describe the syntax of multiple inheritance. When do we use such an inheritance?
Syntax:
Class D : Visibility B1, Visibility B2, ... ... ... { (Body of D) }
Then we want of combine several feature to a single class then we use multiple inheritance.
What are the implications of the following two definitions?
- (a) class A: public B, public C(//….);
- (b) class A: public C, public B(//….);
Two are same.
What is a virtual base class?
Whey multiple paths between a bass class and a derived class are exist then this base class is virtual base class. A base class can be made as virtual just adding ‘virtual’ keyword before this base class.
When do we make a class virtual?
To avoid the duplication of inherited members due to multiple paths between base and derived classes we make base class virtual.
What is an abstract class?
An abstract class is one that is not used to create objects.
In what order are the class constructors called when a derived class object is created?
According to the order of derived class header lines
Class D is derived from class B. The class D does not contain any data members of its own. Does the class D require constructors? If yes, why?
D does not require any construct or because a default constructor is always set to class by default.
What is containership? How does it differ from inheritance?
Containership is another word for composition. That is, the HAS-A relationship where A has-a member that is an object of class B.
Difference : In inheritance the derived class inherits the member data and functions from the base class and can manipulate base public/protected member data as its own data. By default a program which constructs a derived class can directly access the public members of the base class as well. The derived class can be safely down cast to the base class, because the derived is-a” base class.
Container : a class contains another object as member data. The class which contains the object cannot access any protected or private members of the contained class(unless the container it was made a friend in the definition of the contained class).The relationship between the container and the contained object is “has-a” instead of “is-a”
Describe how an object of a class that contains objects of other classes created?
By inheriting an object can be created that contains the objects of other class.
Example :
class A { int a; public: void dosomething(); }; class B: class A { int b; public: void donothing(); }; Now if object of B is created ; then if contains: 1. void dosomething ( ); 2. int b; 3. void donothing ( );
State whether the following statements are TRUE or FALSE:
- (a) Inheritance helps in making a general class into a more specific class.
- (b) Inheritance aids data hiding.
- (c) One of the advantages of inheritance is that it provides a conceptual framework.
- (d) Inheritance facilitates the creation of class libraries.
- (e) Defining a derived class requires some changes in the base class.
- (f) A base class is never used to create objects.
- (g) It is legal to have an object of one class as a member of another class.
- (h) We can prevent the inheritance of all members of the base class by making base class virtual in the definition of the derived class.
- (a) TRUE
- (b) FALSE
- (c) TRUE
- (d) TRUE
- (e) FALSE
- (f) TRUE
- (g) TRUE
- (h) FALSE
Next Previous