Chapter 3: Review Questions
Enumerate the rules of naming variables in C++. How do they differ from ANSI C rules?
Rules of naming variables in C++ are given below :
- a. Any character from ‘a’ to ‘z’ or ‘A’ to ‘Z’ can be used.
- b. Digit can be used but not at the beginning.
- c. Underscore can be used but space is not permitted.
- d. A keyword cannot be used as variable name.
- In C++, a variable can be declared any where in the program but before the using of the variable.
- In C all variables must be declared at the beginning of the program.
An unsigned int can be twice as large as the signed int. Explain how?
In case of unsigned int the range of the input value is : 0 to 2m – 1. [where m is no. of bit]
In case of signed int the range of the input value is : -2m–1 to + (2m–1 – 1)
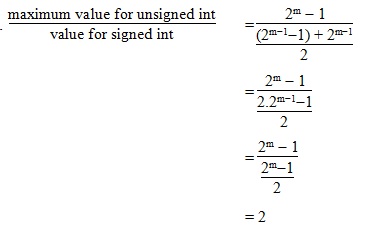
So, maximum value for unsigned int can be twice as large as the signed int.
* Here the absolute value of lower value -2m–1 for signed int must be considered for finding average value of signed int.
Why does C++ have type modifiers?
To serve the needs of various situation more precisely.
What are the applications of void data type in C++?
Two normal uses of void are
- (1) to specify the return type of a function when it is not returning any value.
- (2) To indicate any empty argument list to a function.
Example : void function (void)
Another interesting use of void is in the declaration of generic pointers.
Example :
void *gp; //gp is generic pointer.
A pointer value of any basic data type can be assigned to a generic pointer
int * ip;
gp = ip; // valid.
Can we assign a void pointer to an int type pointer? If not, why? Now can we achieve this?
We cannot assign a void pointer to an int type pointer directly. Because to assign a pointer to another pointer data type must be matched. We can achieve this using casting.
Example :
void * gp; int *ip; ip = (int * ) gp;
Describe, with examples, the uses of enumeration data types.
An enumerated data type is a user-defined type. It provides a way for attaching names to numbers in ANSIC
Example :
enum kuet (EEE, CSE, ECE, CE, ME, IEM);
The enum keyword automatically enumerates
EEE to 0
CSE to 1
ECE to 2
CE to 3
ME to 4
IEM to 5
In C++ each enumerated data type retains its own separate type.
Describe the differences in the implementation of enum data type in ANSI C and C++.
Consider the following example :
enum kuet (EEE, CSE, ECE, CE, ME, IEM);
Here, kuet is tag name.
In C++ tag name become new type name. We can declare a new variables Example:
kuet student;
ANSI C defines the types of enum to be int.
In C int value can be automatically converted to on enum value. But in C++ this is not permitted.
Example:
student cgp = 3.01 // Error in C++
// OK in C.
student cgp = (student) 3.01 //OK in C++
Why is an army called a derived data type?
Derived data types are the data types which are derived from the fundamental data types. Arrays refer to a list of finite number of same data types. The data can be accessed by an index number from o to n. Hence an array is derived from the basic date type, so array is called derived data type.
The size of a char array that is declared to store a string should be one larger than the number of characters in the string. Why?
An additional null character must assign at the end of the string that’s why the size of char array that is declared to store a string should be one larger than the number of characters in the string.
The const was taken from C++ and incorporated in ANSI C, although quite differently. Explain
In both C and C++, any value declared as const cannot be modified by the program in any way. However there are some differences in implementation. In C++ we can use const in a constant expression, such as const int size = 10; char name [size];
This would be illegal in C. If we use const modifier alone, it defaults to int. For example, const size = 10; means const int size = 10;
C++ requires const to be initialized. ANSI C does not require an initialization if none is given, it initializes the const to o. In C++ a const is local, it can be made as global defining it as external. In C const is global in nature , it can be made as local declaring it as static.
How does a constant defined by cowl differ from the constant defined by the preprocessor statement %define?
Consider a example : # define PI 3.14159
The preprocessor directive # define appearing at the beginning of your program specifies that the identifier PI will be replace by the text 3.14159 throughout the program. The keyword const (for constant) precedes the data type of a variable specifies that the value of a variable will not be changed throughout the program.
In short, const allows us to create typed constants instead of having to use # define to create constants that have no type information.
In C++. a variable can be declared anywhere in the scope. What is the significance of this feature?
It is very easy to understand the reason of which the variable is declared.
What do you mean by dynamic initialization of a variable? Give an example.
When initialization is done at the time of declaration then it is know as dynamic initialization of variable
Example :
float area = 3.14159*rad * rad;
What is a reference variable? What is its major use?
A reference variable provides an alias (alternative name) for a previously defined variable.
A major application of reference variables is in passing arguments to functions.
List at least four new operators added by C++ which aid OOP.
New opperators added by C++ are :
- 1. Scope resolution operator ::
- 2. Memory release operator delete   delete
- 3. Memory allocation operator   new
- 4. Field width operator   setw
- 5. Line feed operator   endl
What is the application of the scope resolution operator :: in C++?
A major application of the scope resolution operator is in the classes to identify the class to which a member function belongs.
What are the advantages of using new operator as compared to the junction ntalloc()
Advantages of new operator over malloc ():
- 1. It automatically computes the size of the data object. We need not use the operator size of.
- 2. It automatically returns the correct pointer type, so that there is no need to use a type cast.
- 3. It is possible to initialize the object while creating the memory space.
- 4. Like any other operator, new and delete can be overloaded.
Illustrate with an example, how the seize manipulator works.
setw manipulator specifies the number of columns to print. The number of columns is equal the value of argument of setw () function.
For example:
setw (10) specifies 10 columns and print the massage at right justified.
cout << set (10) << “1234”; will print
1 | 2 | 3 | 4 |
If argument is negative massage will be printed at left justified.
cout <<setw(-10)<< “1234”; will print
1 | 2 | 3 | 4 |
Next Previous